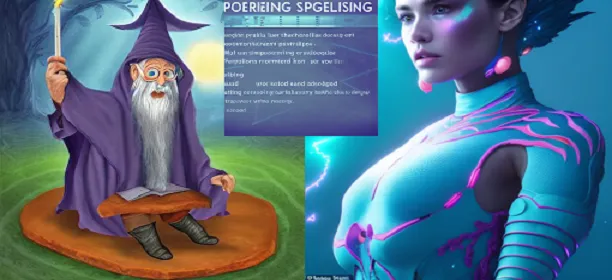
2023
Programming paradigms
Programming Paradigms - Overview, Definitions and Benefits with Examples. Some advantages of the functional paradigm over others.
The programming paradigms are the fundamental style of programming. They serve as a model for the overall creation of a program project (consisting of one or more individual programs).
The programming paradigms overview
Traditional grammar of Latin, Greek, and other inflected languages presents the paradigm as a table of all the inflected forms of a particular verb, noun, or adjective, serving as a model for other words of the same conjugation or declension.
The programming paradigm provides (and determines) how the programmer views program execution. For example, in the object-oriented programming paradigm, the programmer may view the program as a set of interacting objects, while in the functionally oriented paradigm, the program may be viewed as a sequence of calculations of functions that do not have their own states.
Different programming languages recommend different programming paradigms. Some languages are designed to support a specific programming paradigm – SmallTalk and Java support object-oriented programming, while Lisp supports functional, procedural, reflective, meta programming paradigms. The C ++ language supports object-oriented programming and procedural programming.
Most programming languages support more than one programming paradigm to allow programmers to use the most suitable programming style and associated language constructs for a given project.
Imperative paradigm
Imperare / Latin / – order, command is a paradigm in programming, according to which a program consists of a series of commands that determine what and in what order the computer should do.
Imperative programming is the oldest paradigm in programming. Examples of programming languages using this paradigm are ALGOL, Fortran, Pascal, ADA, PL / I, Cobol, C and assembly languages.
C program to display "Hello world", using imperative paradigm
#include <stdio.h> int main() { // The imperative command printf("Hello, world"); return 0; }
Procedural paradigm
Procedural programming is a programming paradigm, derived from imperative programming, based on the concept of the procedure call. Procedures (a type of routine or subroutine) simply contain a series of computational steps to be carried out. Any given procedure might be called at any point during a program’s execution, including by other procedures or itself. The first major procedural programming languages appeared circa 1957–1964, including Fortran, ALGOL, COBOL, PL/I and BASIC. Pascal and C were published circa 1970–1972.
C++ program to display "Hello world", using procedural paradigm
#include <iostream> using namespace std; // The procedure void DoGreetings() { cout << "Hello world"; } int main() { DoGreetings(); return 0; }
Object-oriented paradigm
Object-oriented programming it is to break down a programming task into objects that expose behavior (methods) and data (members or attributes) using interfaces. The most important distinction is that while procedural programming uses procedures to operate on data structures, object-oriented programming bundles the two together, so an "object", which is an instance of a class, operates on its "own" data structure.
Nomenclature varies between the two, although they have similar semantics:
Procedural Object-oriented
Procedure – Method
Record – Object
Module – Class
Procedure call – Message
C Sharp program to display "Hello world", using object-oriented paradigm
The programming styles described above are derived from the imperative (procedural) paradigm. Characteristic of this paradigm is to show the sequence of actions in the processing of data to obtain the final result. The decision comes down to describing HOW to get the result.
The languages supporting these paradigms are related to the architecture of modern computers calls Neumann by the name of its creator – John von Neumann.
In descriptive languages, the programming paradigm is different – the program describes the properties of the desired result but does not show the way to achieve it. Descriptive languages describe WHAT is calculated, not HOW. These are: LISP, PROLOG, POP-11, TABLOG and others.
The main feature of this type of software systems, which distinguishes them from standard software systems, is the use of knowledge in them. The knowledge in these systems is presented with the help of “freer” formalisms (rules, semantic networks, frames, etc.) in contrast to traditional software systems, where knowledge is presented in the form of algorithms, i.e., highly formalized.
Functional paradigm
In computer science, functional programming is a programming paradigm where programs are constructed by applying and composing functions. It is a declarative programming paradigm in which function definitions are trees of expressions that map values to other values, rather than a sequence of imperative statements which update the running state of the program.
In functional programming, functions are treated as first-class citizens, meaning that they can be bound to names (including local identifiers), passed as arguments, and returned from other functions, just as any other data type can. This allows programs to be written in a declarative and composable style, where small functions are combined in a modular manner.
Lisp program to display "Hello world", using functional paradigm
Functional programming allows us to express our code more clearly. We can write less lines of code to achieve what we need.
In the case of Lisp, we have the ability to use functions as data structures, which means we can pass them around instead of having to create new objects every time.
Conclusion
The programming paradigms are the fundamental style of programming
- Object-oriented paradigm formats data as classes. Classes can create objects (class instances). Objects have properties and methods.
- Functional paradigm calculates the estimate of mathematical functions and avoids state-changing inconsistent data.
There are several basic programming paradigms
– Imperative paradigm.
– Procedural paradigm.
– Object-oriented paradigm.
– Functional paradigm.
Learn more about PHP debug code and Sorting algorithms.
Contact
Missing something?
Feel free to request missing tools or give some feedback using our contact form.
Contact Us