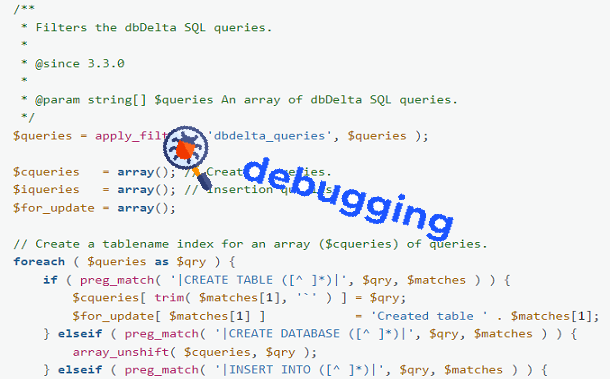
2023
Easily find errors on a WordPress website and debug PHP code
Some simple and easy methods to debug PHP code and detect errors in a WordPress page. Use the built-in PHP functions to debug PHP code.
On the surface, debugging WordPress page or debug PHP code on any other page can be super-duper intimidating. But don't worry mate! This simple methods will help you to cope with the work much easier. Adding this to any page will turn on PHP errors that were previously hidden and display them in a manner you can actually read.
Debug PHP code in WordPress pages
The default user roles in WordPress:
- Subscriber
- Contributor
- Author
- Editor
- Administrator
Naturally, the Administrator has the rights over the entire system. We will make a script that allows the Administrator to review each page for errors without affecting other users. You can test the simple solution by passing it inside the functions.php file of your theme, put it to the header, and print errors, warnings and notices:
add_action('wp_head', 'debug_current_page'); function debug_current_page() { if (is_user_logged_in()) { $user = wp_get_current_user(); $roles = (array) $user->roles; if (in_array('administrator', $roles)) { print_r($roles); ini_set(display_startup_errors, 1); ini_set('display_errors', true); ini_set('html_errors', true); error_reporting(E_ALL); } } }
Once the function is added, visit any website page and check for the roles and debug messages.
Debug PHP code of any web page
Diagnosing a particular PHP page is even easier if it resides outside of a content management system like WordPress. Place the code at the top of the page to show the errors.
<?php ini_set("display_startup_errors", 1); ini_set("display_errors", true); ini_set("html_errors", true); error_reporting(E_ALL); /* Your PHP code below. */ ?>
What really these built-in PHP functions do?
The ini_set() function overrides the directives in php.ini file. This file stores important configuration settings (directives) of the PHP language, such as the amount of RAM allowed to be used, the duration of the script, the time zone, etc. You can find the full list of PHP directives on the official PHP website.
The display_startup_errors will display errors that occur during PHP's startup sequence. It's recommended to enable display_startup_errors for debugging.
The display_errors directive set to true will display the errors to the user. The dispay_errors directive is set to true during development stage of any software project.
The last directive html_errors will display any syntax errors such as missing quotation marks or braces.
The error_reporting(E_ALL) sets the error level of which PHP errors are reported and in our case the debug code will display all PHP errors.
Conclusion
Sometimes it is possible that all the checking functions do not detect errors, but the result of executing the code is wrong. Check the scope of PHP variables. It is possible to expect a result from a global variable without declaring it as such. If you are using Zend Framework pay attention to the init() and preDispatch() methods. The method preDispatch() is called for instances of Zend_Controller_Plugin_Abstract. The method init() of the Zend_Controller_Action is called next as part of the constructor. You can also use PHP extensions for debugging like Xdebug, which provides features to improve the PHP development process.
Learn more about WordPress content type.
Contact
Missing something?
Feel free to request missing tools or give some feedback using our contact form.
Contact Us